I was recently asked to investigate how quickly aggregate medical content from various sources in a portal environment. One path I explored was to use Adobe Flex as front-end technology and access remote web services such as the ones offered by ICW LifeSensor.
With Flex 3.0 you can easily use a Web Service directly from your MXML or ActionScript code, including .NET based web services.
However there are some restrictions. For security reasons, applications running in Flash Player on client computers can only access remote data sources if one of the following conditions is met:
The good news is that BlazeDS, in addition to add RPC capabilities to Flex, acts as a Proxy, so won't have to write my own!
Installing and trying BlazeDS
BlazeDS is really easy to install. I choose to download the turnkey version to start because it includes a runtime environment (Apache Tomcat) and lot of samples.
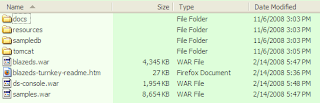
After download and unzip, the only things I had to do was to start the database for the samples (Hypersonic/HSQLDB) and start tomcat and point to http://localhost:8400/samples/. The "Take the test drive" of the tutorial contains a section (sample 2) using web services. The sample code is accessible in .\blazeds_turnkey_3-0-0-544\tomcat\webapps\samples\testdrive-webservice\src\main.mxml:
With Flex 3.0 you can easily use a Web Service directly from your MXML or ActionScript code, including .NET based web services.
However there are some restrictions. For security reasons, applications running in Flash Player on client computers can only access remote data sources if one of the following conditions is met:
- Your SWF file is in the same domain as the remote data source.
- A cross-domain policy file is installed on the web server hosting the data source.
- You use a proxy and your SWF file is on the same server as the proxy.
The good news is that BlazeDS, in addition to add RPC capabilities to Flex, acts as a Proxy, so won't have to write my own!
Installing and trying BlazeDS
BlazeDS is really easy to install. I choose to download the turnkey version to start because it includes a runtime environment (Apache Tomcat) and lot of samples.
After download and unzip, the only things I had to do was to start the database for the samples (Hypersonic/HSQLDB) and start tomcat and point to http://localhost:8400/samples/. The "Take the test drive" of the tutorial contains a section (sample 2) using web services. The sample code is accessible in .\blazeds_turnkey_3-0-0-544\tomcat\webapps\samples\testdrive-webservice\src\main.mxml:
<mx:Application xmlns:mx="http://www.adobe.com/2006/mxml" backgroundColor="#FFFFFF">
<mx:WebService id="srv" destination="ws-catalog" useProxy="true" showBusyCursor="true"/>
<mx:DataGrid dataProvider="{srv.getProducts.lastResult}" width="100%" height="100%">
<mx:columns>
<mx:DataGridColumn dataField="productId" headerText="Product Id"/>
<mx:DataGridColumn dataField="name" headerText="Name"/>
<mx:DataGridColumn dataField="price" headerText="Price"/>
</mx:columns>
</mx:DataGrid>
<mx:Button label="Get Data" click="srv.getProducts()"/>
</mx:Application>
The destination of the web service is defined in .\samples\WEB-INF\flex\proxy-config.xml:<destination id="ws-catalog"> <properties> <wsdl>http://livecycledata.org/services/ProductWS?wsdl</wsdl> <soap>*</soap> </properties> <adapter ref="soap-proxy"/> </destination> Installing and trying BlazeDS The next step for me was to try to build a new Flex application from scratch that uses a web service from an outside domain. I decided to use one of my favorite free testing Web service, the "CDS - Centre de Données astronomiques de Strasbourg" located in Alsace, France which provides access to Astronomical data, including Messier Objects. Sesame is one of the apache axis based services hosted by CDS. In the same way, this new service is declared in the proxy-config.xml file:<destination id="ws-sesame"> <properties> <wsdl>http://cdsws.u-strasbg.fr/axis/services/Sesame?wsdl</wsdl> <soap>*</soap> </properties> <adapter ref="soap-proxy"/> </destination> The Flex program is very easy to construct. I have a list of Messier Objects in a combo box with their IDs (Mxxxx) that are passed as argument for the SesameXML web service operation (see WDSL file). The web service call indicated that BlazeDS is used as as proxy (useProxy="true") and define two ActionScripts methods to handle the result coming back from the remote service and to handle any error (respectively getData_result and getData_fault).
<?xml version="1.0" encoding="utf-8"?> <mx:Application xmlns:mx="http://www.adobe.com/2006/mxml" layout="absolute"> <mx:Script> <![CDATA[ import mx.controls.Alert; import mx.rpc.events.ResultEvent; import mx.rpc.events.FaultEvent; import mx.utils.ObjectUtil; // This software uses source code created at the Centre de Données astronomiques de Strasbourg, France. private function getData():void { webService.SesameXML.send();} private function getData_result(evt:ResultEvent):void {textArea.text = ObjectUtil.toString(evt.result);} private function getData_fault(evt:FaultEvent):void {Alert.show(evt.type);} ]]> </mx:Script> <mx:WebService id="webService" destination="ws-sesame" useProxy="true"> <mx:operation name="SesameXML" resultFormat="object" result="getData_result(event);" fault="getData_fault(event);"> <mx:request> <name>{messier_object.selectedItem.data}</name> </mx:request> </mx:operation> </mx:WebService> <mx:ApplicationControlBar dock="true"> <mx:Button id="button" label="get Messier Data" click="getData();" /> <mx:Spacer width="10%"/> <mx:ComboBox id="messier_object" width="200"> <mx:dataProvider> <mx:ArrayCollection> <mx:source> <mx:Object label="Crab Nebula" data="M1"/> <mx:Object label="Butterfly Cluster" data="M6"/> <mx:Object label="Butterfly Cluster" data="M6"/> <mx:Object label="Ptolemy Cluster" data="M7"/> <mx:Object label="Lagoon Nebula" data="M8"/> <mx:Object label="Wild Duck Cluster" data="M11"/> <mx:Object label="Great Globular Cluster in Hercules" data="M13"/> <mx:Object label="Eagle Nebula" data="M16"/> <mx:Object label="Omega Nebula" data="M17"/> <mx:Object label="Trifid Nebula" data="M20"/> <mx:Object label="Sagittarius Cluster" data="M22"/> <mx:Object label="Sagittarius Star Cloud" data="M24"/> </mx:source> </mx:ArrayCollection> </mx:dataProvider> </mx:ComboBox> </mx:ApplicationControlBar> <mx:TextArea id="textArea" editable="false" width="100%" height="100%" /> </mx:Application>Building the SWF file
To build your shockwave executable file, it is important to indicate where the services configuration file is located, so the BlazeDS stub is added to the *.SWF file running in the browser inside the Flash Player and will make the connection of the BlazeDS proxy.
In fact, services-config.xml describes the different services that the web application is using:mxmlc -strict=true \ -show-actionscript-warnings=true \ -use-network=true \ -services=WEB-INF/flex/services-config.xml \ -context-root=samples \ -output=testdrive-webservice/main.swf testdrive-webservice/src/main.mxm
<services-config> <services> <service-include file-path="proxy-config.xml" /> ... </services> .... </services-config>You will also need to modify your ./docroot/WEB-INF/web.xml file by adding the definitions of the listener and the MessageBroker Servlet definition.<web-app> <display-name>WebTest</display-name> <description>Application with Samples</description> <context-param> <param-name>flex.class.path</param-name> <param-value>/WEB-INF/flex/hotfixes</param-value> </context-param> <!-- Http Flex Session attribute and binding listener support --> <listener> <listener-class>flex.messaging.HttpFlexSession</listener-class> </listener> <!-- MessageBroker Servlet --> <servlet> <servlet-name>MessageBrokerServlet</servlet-name> <display-name>MessageBrokerServlet</display-name> <servlet-class>flex.messaging.MessageBrokerServlet</servlet-class> <init-param> <param-name>services.configuration.file</param-name> <param-value>/WEB-INF/flex/services-config.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>MessageBrokerServlet</servlet-name> <url-pattern>/messagebroker/*</url-pattern> </servlet-mapping> </web-app>Here is the result after querying information about Messier object (M1 - Crab Nebula):
The next task for me to see how to integrate BlazeDS in a portal environment such as Liferay and explore authentication to Web Services and security features associated to the use of BlazeDS.
No comments:
Post a Comment